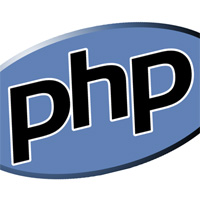
Simple Server-Side Browser Detection with PHP
Ever wish you could make a webpage behave according to the browser it is being viewed with? In many cases this can be done with some well-placed CSS code. But, how about those situations when CSS can’t help us out? CSS hacks and tricks won’t let you target Firefox, Chrome or mobile devices. So, do you just stay away from using custom content in those browsers?
Enter PHP browser detection! With just a little bit of code, your site can reach its maximum potential in every browser, and even in mobile devices.
Some common uses for browser detection include:
- – avoiding choppy JavaScript transitions by serving different versions of the script according to browser or device performance
- – serving alternate content to iPhone browsers where Flash would otherwise be used
- – getting rid of elements that cause rendering problems in some browsers but aren’t essential
- – automatically populating a form with the current browser and version for simple debugging purposes
To get an idea of usage, load this page in Chrome or Safari and take a look at the header. Now load it in Internet Explorer. Those subtle differences you see in the header allow the code to run more smoothly in mobile devices and IE. And though it doesn’t look exactly the same, I’ve had no problems with branding the recognizable animation as my handle. Enough talk — let’s get to it, then.
The Basic Example Code
Here is an implementation of this code as it appears in the header.php of this site:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | <!-- Top Menu --> <div id="logo"><a href="<?php bloginfo('url'); ?>"><img src="<?php bloginfo('template_directory'); ?>/images/logo.png" /></a></div> <?php $user_agent = $_SERVER['HTTP_USER_AGENT']; if (preg_match('/iphone/i', $user_agent)) wp_nav_menu( array('menu' => 'topnavie' )); elseif (preg_match('/MSIE/i',$u_agent) && !preg_match('/Opera/i',$u_agent)) wp_nav_menu( array('menu' => 'topnavie' )); } else { wp_nav_menu( array('menu' => 'topnav' )); print '<a href="https://www.dauid.us/wp-content/uploads/2010/12/dauidus1" target="_blank"><div id="bird"></div></a>'; }; ?> |
As you can see here, I’m using a single line of code that tells the server to check the user agent. Then we just employ normal PHP if statements to define what should be displayed in each browser. First, I check for both the iPhone and IE and serve a stripped-down version of my site menu. Then, I serve everything else a more beefed-up version of that same menu. Of course, this is all pretty basic so far.
Going a Bit Deeper
But what if you need to target other browsers? Here’s the code to target some other common browsers without any additional formatting:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | <?php // check what browser the visitor is using $user_agent = $_SERVER['HTTP_USER_AGENT']; // This bit differentiates IE from Opera if(preg_match('/MSIE/i',$u_agent) && !preg_match('/Opera/i',$u_agent)) { print 'This is Internet Explorer. (Insert joke here)'; } elseif(preg_match('/mozilla/i',$u_agent) && !preg_match('/compatible/', $userAgent)) { print 'This is FireFox.'; } // let Chrome be recognized as Safari elseif(preg_match('/webkit/i',$u_agent)) { print 'This is either Chrome or Safari.'; } elseif(preg_match('/Opera/i',$u_agent)) { print 'This is Opera. Like to live on the edge, huh?'; } elseif(preg_match('/Netscape/i',$u_agent)) { print 'This is Netscape, and you really need to upgrade.'; } ?> |
Now, there are a few things to be explained here, even before we really get into the code. The opera browser has had many problems in the past regarding user agent spoofing. This spoofing was necessary because the MSN and IE browsers allowed developers to only serve content for their software (which was, at the time, considered to be top-notch). Pretty much, this was IE’s attempt at sabotaging the web into using their browser out of necessity, not choice (tisk, tisk, IE). In order for that content to be visible in Opera, their developers found it necessary to spoof their user agent to look and act like IE. Now, this really hasn’t been too much of a problem since 2005, when Opera 8.02 was released with the option to use its own user agent string (and finally grew up as a big-boy browser… sort-of) But as developers, we must remember that content needs to be served to the widest possible audience (as some companies won’t allow their employees to upgrade software).
So, why did I make you sit through that winded explanation? Take a look again at that first line of code above. Notice that we not only detect the IE browser, but also conditionally detect for “if not opera.” This should protect against all that nasty user agent spoofing for those who run versions of Opera younger than 8.02. But, that’s not all. Notice that we also later detect for the opera user agent with no other conditionals. This should take care of all those later versions of Opera which don’t run into the spoofing stuff.
Still with me? Good, because we’re not quite done with the explanations. It’s not really spoofing, but Chrome and Safari both use the ‘webkit’ user agent (very familiar to anyone whose written CSS3). So, using our script, Chrome will always be recognized as Safari (the default webkit browser). This really shouldn’t be a problem if you are just wanting your content to be served identically across browsers. But, if you’re looking to provide different content for those two browsers, this solution will not work for you.
Detecting Browser Versions
So, what about those instances when you really need to serve alternate content to specific versions of browsers (can you say IE6)?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | <?php // check what browser the visitor is using $user_agent = $_SERVER['HTTP_USER_AGENT']; // This bit differentiates IE from Opera if(preg_match('/(?i)MSIE [1-6]/',$u_agent) && !preg_match('/Opera/i',$u_agent) && ) { // if IE<=6 print 'This is IE6 or less (the browsers of infamous dooooom!)'; } else { // if IE>6 print 'This is IE7 or greater.'; } ?> |
So, what we’ve done here is added the major versions to our ‘preg_match’ detection string. In this case, we will check for IE versions 1-6 and serve some content for those. If that doesn’t match the version, we can assume the user is on IE7 or above and serve them alternate content for those browser versions.
So there you have it… simple browser detection using nothing but a few lines of PHP. In a small number of cases, this script can produce false positives (for example, if IE is being run in compatibility mode). In those cases, it may be best to run your site through a CMS and use a pre-packaged plugin. I know there are a few for WordPress, but most have far more code than is necessary for most uses. If you have found one of these plugins, let me know about it in the comments.
Caio!
Dave, can you help me with this code? I’m trying to edit it to work with Firefox, but it’s proving difficult.
In IE the version is displayed like this in the User-Agent (up to version 9):
MSIE 9.0
Whereas in FF it’s like:
Firefox/36.0
The space is replaced with a / so even if I change MSIE to Firefox in the preg_match function above it won’t work.
This is the code:
preg_match(‘/MSIE (.*?);/’, $_SERVER[‘HTTP_USER_AGENT’], $matches);
echo $matches[1];
John,
Try this:
Browser sniffing can be done in many ways… I’ve only provided one example in my post.
Hope it helps.
-D
I’m impressed that this is possible with PHP. I’m new to WP, and I’m looking for a way to change some things on my site depending on the browser. It seems like its possible. But, how does it work? Is there a plugin or do I have to put the code in myself? Thanks for helping.
Brenda,
This code must be placed directly into the corresponding theme file. So, if you wanted to change your site’s logo based on the browser, you would simply amend the code above to where you would like your logo to appear, then just change out the logos. It gets a bit more complicated when you try to add supreme functionality. I’m sure you could find some other resources on the web that help you out with this… and I think there are a few WordPress plugins, too. Holla’ back if you need any more help.
-Dave
great job, I like your blog